Android added this functionality already, but for my target deployment I had to implement it from scratch . I usually end up doing that anyway. So the idea is to have a login form with username / password / login button and next to the password field you would have a small button that toggles password obfuscation on and off. It looks like this:
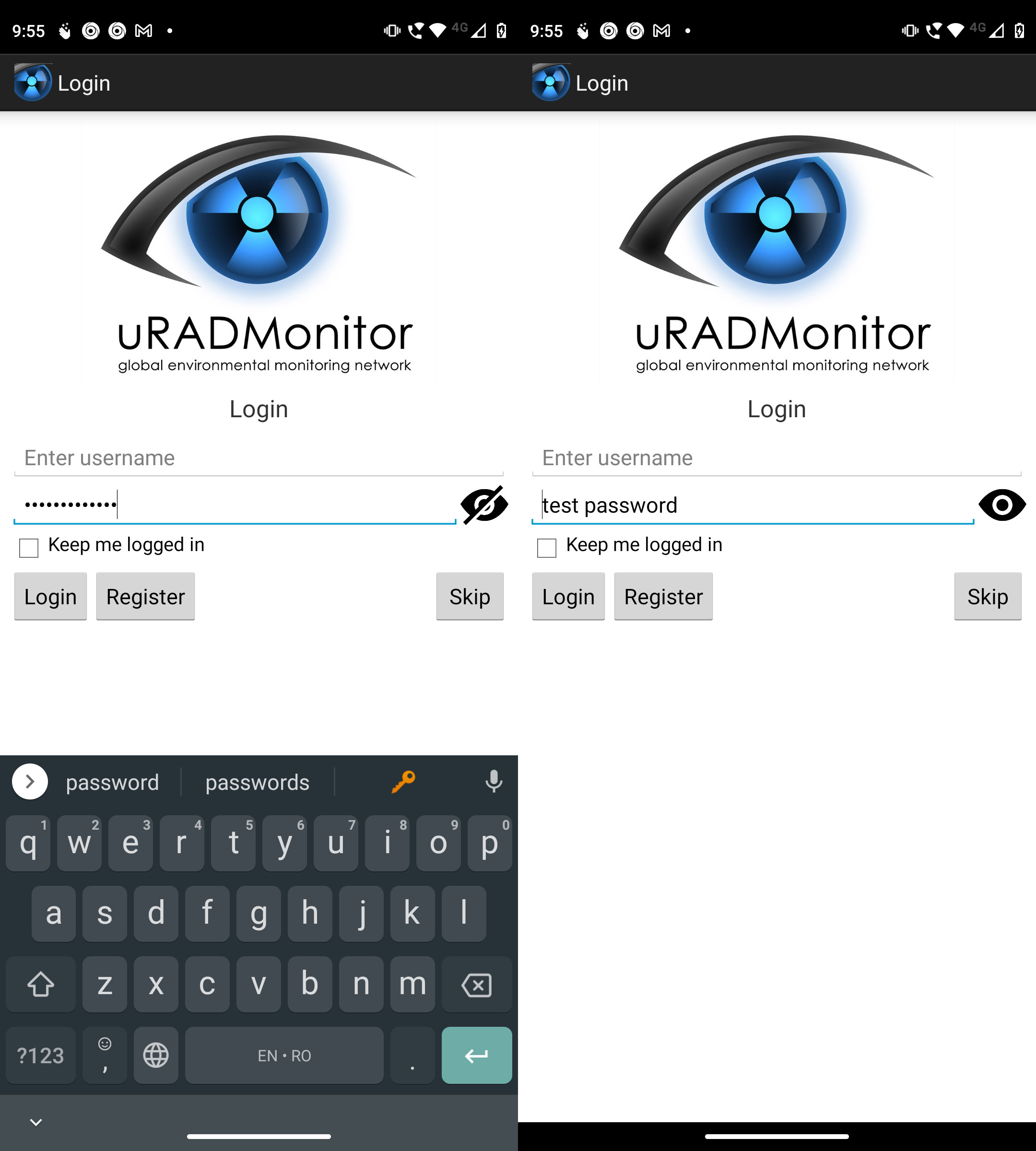
The implementation is trivial. You need to keep the current state so you can implement the toggle. This state can be anything, I used the transformation property of the password editbox and changed button / transformation against that. Here’s the code:
LinearLayout hl = new LinearLayout(this);
hl.setOrientation(LinearLayout.HORIZONTAL);
content.addView(hl);
final EditText etp = new EditText(this);
etp.setHint(R.string.hint_password);
etp.setTransformationMethod(new PasswordTransformationMethod());
hl.addView(etp, new LinearLayout.LayoutParams(LayoutParams.MATCH_PARENT, LayoutParams.WRAP_CONTENT, 1.0f));
final ImageView iv = new ImageView(this);
iv.setAdjustViewBounds(true);
iv.setMaxHeight(100);
iv.setImageResource(R.drawable.hide);
hl.addView(iv);
iv.setOnClickListener(new OnClickListener() {
@Override
public void onClick(View v) {
if (etp.getTransformationMethod() == null) {
iv.setImageResource(R.drawable.hide);
etp.setTransformationMethod(new PasswordTransformationMethod());
} else {
iv.setImageResource(R.drawable.show);
etp.setTransformationMethod(null);
}
}
});
The right button (eye symbol) works as a toggle switch and will show / hide the password content. This is a very useful feature when entering passwords, to do a quick check before hitting that Login button and avoid any typos.